Vue Js Intersection of Two Array: To get the intersection of two arrays of objects in Vue.js using filter and some, we can iterate over one of the arrays and use the some method to check if the current object exists in the other array.
First, we can use the filter method to iterate over the first array and return only the objects that have a matching object in the second array using the some method. We pass a function to the some method that checks if any object in the second array has the same id as the current object in the first array.
Implement Intersection of two Array
This code uses Vue.js to display a list of items that are present in both array1
and array2
.
The intersection
computed property filters array1
based on whether each item’s id
exists in array2
, using the some()
method.
Then, the resulting list is displayed using v-for
to iterate through each item and display its name
property in a list item element.
Vue Js Intersection of Two Array Example
<div id="app">
<ul>
<li v-for="item in intersection" :key="item.id">{{ item.name }}</li>
</ul>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
array1: [
{ id: 1, name: "Alice" },
{ id: 2, name: "Bob" },
{ id: 3, name: "Charlie" }
],
array2: [
{ id: 2, name: "Bob" },
{ id: 4, name: "Dave" }
]
}
},
computed: {
intersection() {
return this.array1.filter(item1 =>
this.array2.some(item2 => item1.id === item2.id)
);
}
}
})
</script>
Output of above example
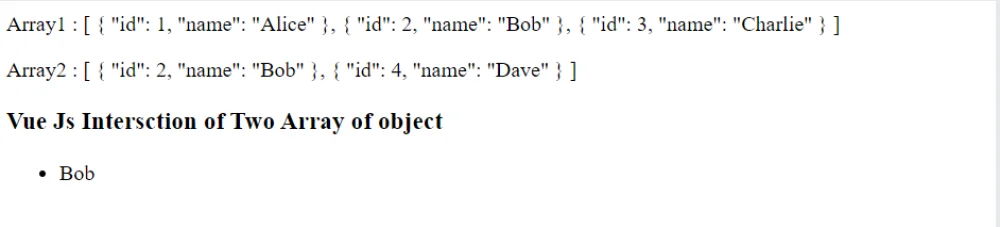
Find the common elements between the Multiple arrays of Object?
This is a Vue.js script that creates a Vue instance with three arrays of objects as data properties. These arrays contain a list of people with unique IDs and names. The script also defines a computed property called “intersection” that computes the common elements between the three arrays using the reduce method and the filter and some array methods. The computed property returns an array of objects that have the same ID value in at least two of the three original arrays. This script can be used to find the common elements between multiple arrays of objects in a Vue application.
Vue Js Intersection of Multiple Array of Object Example
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
arr1: [
{ id: 1, name: "Andrew" },
{ id: 2, name: "Jane" },
{ id: 3, name: "Bob" },
],
arr2: [
{ id: 2, name: "Jane" },
{ id: 3, name: "Bob" },
{ id: 1, name: "Andrew" },
],
arr3: [
{ id: 3, name: "Bob" },
{ id: 4, name: "Alice" },
{ id: 1, name: "Andrew" },
],
}
},
computed: {
intersection() {
const arrays = [this.arr1, this.arr2, this.arr3];
return arrays.reduce((acc, arr) =>
acc.filter((x) => arr.some((y) => y.id === x.id))
);
},
},
})
</script>
Output of Vue Js Intersection of Multiple array of object
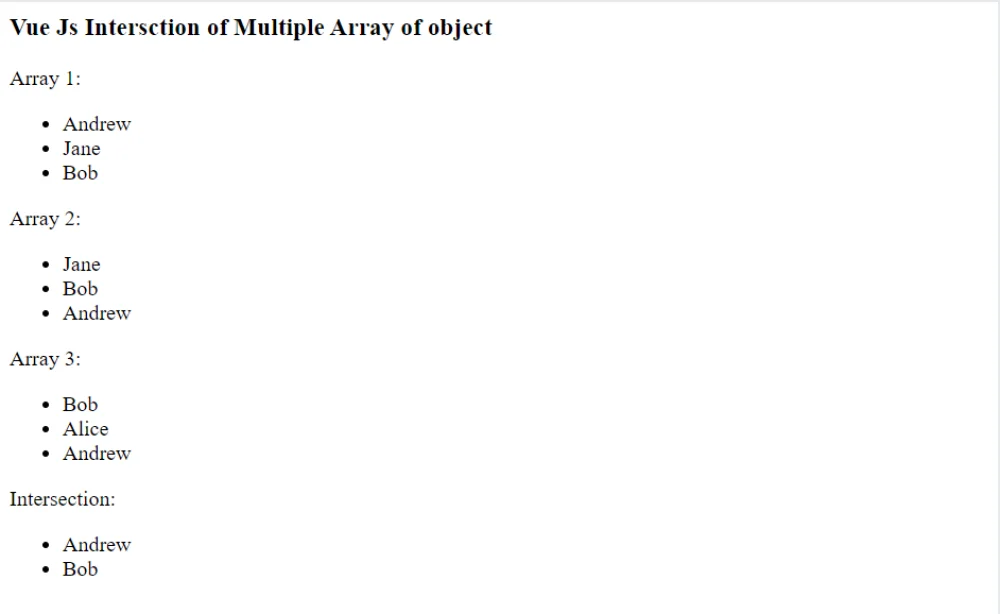